Inverse design optimization of a mode converter#
Note: Tidy3D now supports automatic differentiation natively through
autograd
. Thejax
-basedadjoint
plugin will be deprecated from 2.7 onwards. To see this notebook implemented in the new feature, see this notebook.
To install the
jax
module required for this feature, we recommend runningpip install "tidy3d[jax]"
.
In this notebook, we will use inverse design and the Tidy3D adjoint
plugin to create an integrated photonics component to convert a fundamental waveguide mode to a higher order mode.
[1]:
from typing import List
import numpy as np
import matplotlib.pylab as plt
# import jax to be able to use automatic differentiation
import jax.numpy as jnp
from jax import grad, value_and_grad
# import regular tidy3d
import tidy3d as td
import tidy3d.web as web
from tidy3d.plugins.mode import ModeSolver
# import the components we need from the adjoint plugin
from tidy3d.plugins.adjoint import JaxSimulation, JaxBox, JaxCustomMedium, JaxStructure, JaxStructureStaticGeometry
from tidy3d.plugins.adjoint import JaxSimulationData, JaxDataArray, JaxPermittivityDataset
from tidy3d.plugins.adjoint.web import run
# set random seed to get same results
np.random.seed(2)
Setup#
We wish to recreate a device like the diagram below:
A mode source is injected into a waveguide on the left-hand side. The light propagates through a rectangular region with pixellated permittivity with the value of each pixel independently tunable between 1 (vacuum) and some maximum permittivity. Finally, we measure the transmission of the light into a waveguide on the right-hand side.
The goal of the inverse design exercise is to find the best distribution of permittivities (\(\epsilon_{ij}\)) in the coupling region to maximize the power conversion between the input mode and the output mode.
We also apply our built-in smoothening and binarization filters to ensure that the final device has smooth features, and permitivitty values that are all either 1, or the maximum permittivity of the waveguide material.
Parameters#
First we will define some parameters.
[2]:
# wavelength and frequency
wavelength = 1.0
freq0 = td.C_0 / wavelength
k0 = 2 * np.pi * freq0 / td.C_0
# resolution control
min_steps_per_wvl = 16
# in the design region, we set uniform grid resolution,
# and define the design parameters on the same grid
dl_design_region = 0.01
# space between boxes and PML
buffer = 1.0 * wavelength
# optimize region size
lz = td.inf
lx = 5.0
ly = 3.0
# position of source and monitor (constant for all)
source_x = -lx / 2 - buffer * 0.8
meas_x = lx / 2 + buffer * 0.8
# total size
Lx = lx + 2 * buffer
Ly = ly + 2 * buffer
Lz = 0
# permittivity and width of the input/output waveguide
eps_wg = 2.75
wg_width = 0.7
# random starting parameters between 0 and 1
nx = int(lx / dl_design_region)
ny = int(ly / dl_design_region)
params0 = np.random.random((nx, ny))
# frequency width and run time
freqw = freq0 / 10
run_time = 50 / freqw
Static Components#
Next, we will set up the static parts of the geometry, the input source, and the output monitor using these parameters.
[3]:
waveguide = td.Structure(
geometry=td.Box(size=(2*Lx, wg_width, lz)), medium=td.Medium(permittivity=eps_wg)
)
mode_size = (0, wg_width * 3, lz)
source_plane = td.Box(
center=[source_x, 0, 0],
size=mode_size,
)
measure_plane = td.Box(
center=[meas_x, 0, 0],
size=mode_size,
)
Input Structures#
Next, we write a function to return the pixellated array given our flattened tuple of permittivity values \(\epsilon_{ij}\) using JaxCustomMedium.
We start with an array of parameters between 0 and 1, apply a ConicFilter and a BinaryProjector to create smooth, binarized features.
The JaxStructureStaticGeometry
allows for including a jax
-compatible medium and a non-differentiable Tidy3D geometry.
[4]:
from tidy3d.plugins.adjoint.utils.filter import ConicFilter, BinaryProjector
radius = .120
beta = 50
conic_filter = ConicFilter(radius=radius, design_region_dl=float(lx) / nx)
def filter_project(params, beta, eta=0.5):
"""Apply conic filter and binarization to the raw params."""
params_smooth = conic_filter.evaluate(params)
binary_projector = BinaryProjector(vmin=0, vmax=1, beta=beta, eta=eta)
params_smooth_binarized = binary_projector.evaluate(params_smooth)
return params_smooth_binarized
def get_eps(params, beta):
"""Get the permittivity values (1, eps_wg) array as a funciton of the parameters (0, 1)"""
processed_params = filter_project(params, beta)
return 1 + processed_params * (eps_wg - 1)
def make_input_structures(params, beta) -> List[JaxStructure]:
x0_min = -lx / 2 + dl_design_region / 2
y0_min = -ly / 2 + dl_design_region / 2
input_structures = []
coords_x = [x0_min + dl_design_region * ix for ix in range(nx)]
coords_y = [y0_min + dl_design_region * iy for iy in range(ny)]
coords = dict(x=coords_x, y=coords_y, z=[0], f=[freq0])
eps = get_eps(params, beta=beta).reshape((nx, ny, 1, 1))
field_components = {
f"eps_{dim}{dim}": JaxDataArray(values=eps, coords=coords) for dim in "xyz"
}
eps_dataset = JaxPermittivityDataset(**field_components)
custom_medium = JaxCustomMedium(eps_dataset=eps_dataset)
box = td.Box(center=(0, 0, 0), size=(lx, ly, lz))
custom_structure = JaxStructureStaticGeometry(geometry=box, medium=custom_medium)
return [custom_structure]
Jax Simulation#
Next, we write a function to return a basic JaxSimulation
as a function of our parameter values.
We make sure to add the pixellated JaxStructure
list to input_structures
but leave out the sources and monitors for now as weβll want to add those after the mode solver is run so we can inspect them.
[5]:
def make_sim_base(params, beta) -> JaxSimulation:
input_structures = make_input_structures(params, beta=beta)
design_region_mesh = td.MeshOverrideStructure(
geometry=td.Box(size=(lx, ly, lz)),
dl=[dl_design_region] * 3,
enforce=True,
)
grid_spec=td.GridSpec.auto(
wavelength=wavelength,
min_steps_per_wvl=16,
override_structures=[design_region_mesh],
)
return JaxSimulation(
size=[Lx, Ly, Lz],
grid_spec=grid_spec,
structures=[waveguide],
input_structures=input_structures,
sources=[],
monitors=[],
output_monitors=[],
run_time=run_time,
boundary_spec=td.BoundarySpec.pml(x=True, y=True, z=False),
)
Visualize#
Letβs visualize the simulation to see how it looks
[6]:
sim_start = make_sim_base(params0, beta=1.0)
ax = sim_start.plot_eps(z=0)
plt.show()
WARNING:jax._src.xla_bridge:An NVIDIA GPU may be present on this machine, but a CUDA-enabled jaxlib is not installed. Falling back to cpu.
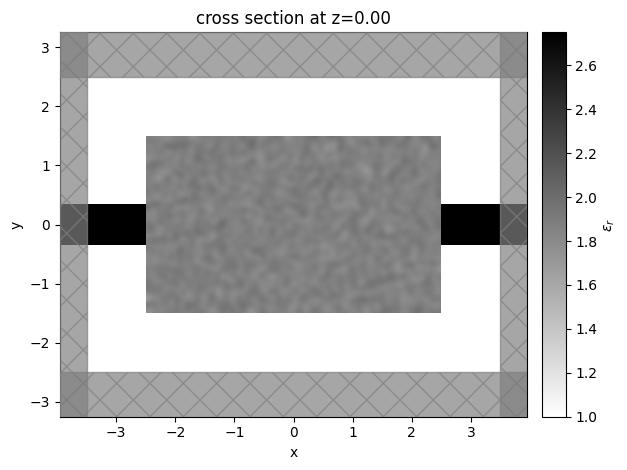
Select Input and Output Modes#
Next, letβs visualize the first 4 mode profiles so we can select which mode indices we want to inject and transmit.
[7]:
from tidy3d.plugins.mode.web import run as run_mode_solver
num_modes = 4
mode_spec = td.ModeSpec(num_modes=num_modes)
mode_solver = ModeSolver(
simulation=sim_start.to_simulation()[0],
plane=source_plane,
mode_spec=td.ModeSpec(num_modes=num_modes),
freqs=[freq0]
)
modes = run_mode_solver(mode_solver, reduce_simulation=True)
11:01:07 -03 WARNING: The associated `Simulation` object contains custom mediums. It will be automatically restricted to the mode solver plane to reduce data for uploading. To force uploading the original `Simulation` object use `reduce_simulation=False`. Setting `reduce_simulation=True` will force simulation reduction in all cases and silence this warning.
11:01:08 -03 Mode solver created with task_id='fdve-4e5fa95b-d57e-438b-903c-2aa81e1b37bf', solver_id='mo-23b407bb-6759-4421-ab18-cf2c9a49dcf0'.
11:01:12 -03 Mode solver status: queued
11:01:29 -03 Mode solver status: running
11:01:38 -03 Mode solver status: success
Letβs visualize the modes next.
[8]:
fig, axs = plt.subplots(num_modes, 3, figsize=(12, 12), tight_layout=True)
for mode_index in range(num_modes):
vmax = 1.1 * max(abs(modes.field_components[n].sel(mode_index=mode_index)).max() for n in ("Ex", "Ey", "Ez"))
for field_name, ax in zip(("Ex", "Ey", "Ez"), axs[mode_index]):
field = modes.field_components[field_name].sel(mode_index=mode_index)
field.real.plot(label="Real", ax=ax)
field.imag.plot(ls="--", label="Imag", ax=ax)
ax.set_title(f'index={mode_index}, {field_name}')
ax.set_ylim(-vmax, vmax)
axs[0, 0].legend()
print("Effective index of computed modes: ", np.array(modes.n_eff))
Effective index of computed modes: [[1.5720801 1.535463 1.3032304 1.1848152]]
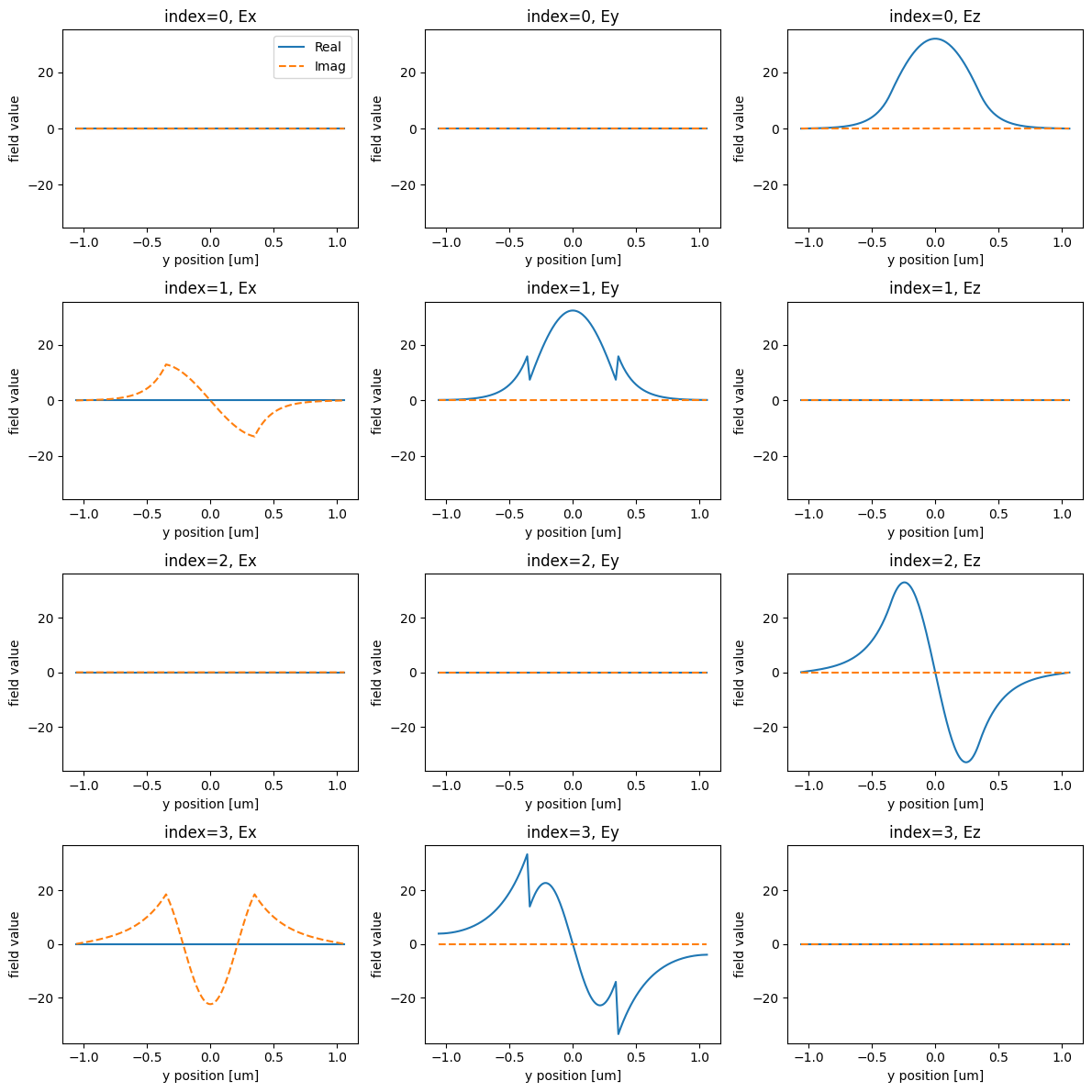
We want to inject the fundamental, Ez-polarized input into the 1st order Ez-polarized input.
From the plots, we see that these modes correspond to the first and third rows, or mode_index=0
and mode_index=2
, respectively.
So we make sure that the mode_index_in
and mode_index_out
variables are set appropriately and we set a ModeSpec
with 3 modes to be able to capture the mode_index_out
in our output data.
[9]:
mode_index_in = 0
mode_index_out = 2
num_modes = max(mode_index_in, mode_index_out) + 1
mode_spec = td.ModeSpec(num_modes=num_modes)
Then it is straightforward to generate our source and monitor.
[10]:
# source seeding the simulation
forward_source = td.ModeSource(
source_time=td.GaussianPulse(freq0=freq0, fwidth=freqw),
center=[source_x, 0, 0],
size=mode_size,
mode_index=mode_index_in,
mode_spec=mode_spec,
direction="+",
)
# we'll refer to the measurement monitor by this name often
measurement_monitor_name = "measurement"
# monitor where we compute the objective function from
measurement_monitor = td.ModeMonitor(
center=[meas_x, 0, 0],
size=mode_size,
freqs=[freq0],
mode_spec=mode_spec,
name=measurement_monitor_name,
)
Finally, we create a new function that calls our make_sim_base()
function and adds the source and monitor to the result. This is the function we will use in our objective function to generate our JaxSimulation
given the input parameters.
[11]:
def make_sim(params, beta):
sim = make_sim_base(params, beta=beta)
return sim.updated_copy(sources=[forward_source], output_monitors=[measurement_monitor])
Post Processing#
Next, we will define a function to tell us how we want to postprocess the output JaxSimulationData
object to give the conversion power that we are interested in maximizing.
[12]:
def measure_power(sim_data: JaxSimulationData) -> float:
"""Return the power in the output_data amplitude at the mode index of interest."""
output_amps = sim_data["measurement"].amps
amp = output_amps.sel(direction="+", f=freq0, mode_index=mode_index_out)
return jnp.sum(jnp.abs(amp) ** 2)
Then, we add a penalty to produce structures that are invariant under erosion and dilation, which is a useful approach to implementing minimum length scale features.
[13]:
from tidy3d.plugins.adjoint.utils.penalty import ErosionDilationPenalty
def penalty(params, beta):
processed_params = filter_project(params, beta=beta)
ed_penalty = ErosionDilationPenalty(length_scale=radius, pixel_size=dl_design_region)
return ed_penalty.evaluate(processed_params)
Define Objective Function#
Finally, we need to define the objective function that we want to maximize as a function of our input parameters (permittivity of each pixel) that returns the conversion power. This is the function we will differentiate later.
[14]:
def J(params, beta: float, step_num: int = None, verbose: bool = False) -> float:
sim = make_sim(params, beta=beta)
task_name = "inv_des"
if step_num:
task_name += f"_step_{step_num}"
sim_data = run(sim, task_name=task_name, verbose=verbose)
penalty_weight = np.minimum(1, beta/25)
return measure_power(sim_data) - penalty_weight * penalty(params, beta)
Inverse Design#
Now we are ready to perform the optimization.
We use the jax.value_and_grad
function to get the gradient of J
with respect to the permittivity of each Box
, while also returning the converted power associated with the current iteration, so we can record this value for later.
Letβs try running this function once to make sure it works.
[15]:
dJ_fn = value_and_grad(J)
[16]:
val, grad = dJ_fn(params0, beta=1, verbose=True)
print(grad.shape)
11:01:49 -03 Created task 'inv_des' with task_id 'fdve-f3b38f0f-34db-4b3f-9513-dd50b621b10b' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-f3b38f0f-34d b-4b3f-9513-dd50b621b10b'.
11:01:55 -03 status = queued
11:02:08 -03 status = preprocess
11:02:12 -03 Maximum FlexCredit cost: 0.025. Use 'web.real_cost(task_id)' to get the billed FlexCredit cost after a simulation run.
starting up solver
11:02:13 -03 running solver
To cancel the simulation, use 'web.abort(task_id)' or 'web.delete(task_id)' or abort/delete the task in the web UI. Terminating the Python script will not stop the job running on the cloud.
11:02:19 -03 early shutoff detected at 12%, exiting.
11:02:20 -03 status = postprocess
11:02:39 -03 status = success
View simulation result at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-f3b38f0f-34d b-4b3f-9513-dd50b621b10b'.
11:02:45 -03 loading simulation from simulation_data.hdf5
11:02:47 -03 Created task 'inv_des_adj' with task_id 'fdve-2cd90a08-899a-49af-acd6-8ab36021f0ae' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-2cd90a08-899 a-49af-acd6-8ab36021f0ae'.
11:02:55 -03 status = queued
11:03:10 -03 status = preprocess
11:03:14 -03 Maximum FlexCredit cost: 0.025. Use 'web.real_cost(task_id)' to get the billed FlexCredit cost after a simulation run.
starting up solver
running solver
To cancel the simulation, use 'web.abort(task_id)' or 'web.delete(task_id)' or abort/delete the task in the web UI. Terminating the Python script will not stop the job running on the cloud.
11:03:22 -03 early shutoff detected at 8%, exiting.
status = postprocess
11:03:31 -03 status = success
11:03:32 -03 View simulation result at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-2cd90a08-899 a-49af-acd6-8ab36021f0ae'.
(500, 300)
[17]:
print(grad)
[[ 1.9115957e-08 2.0341925e-08 2.1002823e-08 ... -5.6986043e-09
-5.3410938e-09 -4.9523634e-09]
[ 2.2382387e-08 2.3751845e-08 2.4416215e-08 ... -6.0140790e-09
-5.7981397e-09 -5.4938458e-09]
[ 2.5903649e-08 2.7416876e-08 2.8085157e-08 ... -6.3867702e-09
-6.3510948e-09 -6.1579835e-09]
...
[ 4.1107819e-07 4.7213894e-07 5.3059080e-07 ... -5.0702891e-07
-4.4837859e-07 -3.8811166e-07]
[ 3.7093525e-07 4.2642949e-07 4.7960219e-07 ... -4.5335065e-07
-4.0046683e-07 -3.4622119e-07]
[ 3.2704909e-07 3.7625156e-07 4.2354606e-07 ... -3.9623021e-07
-3.4959373e-07 -3.0191538e-07]]
Optimization#
We will use βAdamβ optimization strategy to perform sequential updates of each of the permittivity values in the JaxCustomMedium.
For more information on what we use to implement this method, see this article.
We will run 10 steps and measure both the permittivities and powers at each iteration.
We capture this process in an optimize
function, which accepts various parameters that we can tweak.
[18]:
import optax
# hyperparameters
num_steps = 20
learning_rate = 1.0
# initialize adam optimizer with starting parameters
params = np.array(params0)
optimizer = optax.adam(learning_rate=learning_rate)
opt_state = optimizer.init(params)
# store history
Js = []
params_history = [params]
beta_history = []
# gradually increase the binarization strength
beta0 = 1
beta_increment = 1
for i in range(num_steps):
# compute gradient and current objective funciton value
beta = beta0 + i * beta_increment
value, gradient = dJ_fn(params, step_num=i+1, beta=beta)
# outputs
print(f"step = {i + 1}")
print(f"\tbeta = {beta:.4e}")
print(f"\tJ = {value:.4e}")
print(f"\tgrad_norm = {np.linalg.norm(gradient):.4e}")
# compute and apply updates to the optimizer based on gradient (-1 sign to maximize obj_fn)
updates, opt_state = optimizer.update(-gradient, opt_state, params)
params = optax.apply_updates(params, updates)
# cap the parameters
params = jnp.minimum(params, 1.0)
params = jnp.maximum(params, 0.0)
# save history
Js.append(value)
params_history.append(params)
beta_history.append(beta)
power = J(params_history[-1], beta=beta)
Js.append(power)
step = 1
beta = 1.0000e+00
J = -3.9940e-02
grad_norm = 4.8046e-04
step = 2
beta = 2.0000e+00
J = 6.8252e-03
grad_norm = 8.9551e-03
step = 3
beta = 3.0000e+00
J = -5.8742e-02
grad_norm = 9.5530e-03
step = 4
beta = 4.0000e+00
J = -8.3863e-02
grad_norm = 5.8784e-03
step = 5
beta = 5.0000e+00
J = 1.3108e-01
grad_norm = 1.1276e-02
step = 6
beta = 6.0000e+00
J = 3.3037e-01
grad_norm = 1.4994e-02
step = 7
beta = 7.0000e+00
J = 4.2607e-01
grad_norm = 1.9793e-02
step = 8
beta = 8.0000e+00
J = 4.7463e-01
grad_norm = 1.4728e-02
step = 9
beta = 9.0000e+00
J = 6.4065e-01
grad_norm = 1.1329e-02
step = 10
beta = 1.0000e+01
J = 7.2823e-01
grad_norm = 1.0882e-02
step = 11
beta = 1.1000e+01
J = 7.6354e-01
grad_norm = 1.3361e-02
step = 12
beta = 1.2000e+01
J = 7.8878e-01
grad_norm = 1.2873e-02
step = 13
beta = 1.3000e+01
J = 8.3580e-01
grad_norm = 6.2414e-03
step = 14
beta = 1.4000e+01
J = 8.4929e-01
grad_norm = 7.4505e-03
step = 15
beta = 1.5000e+01
J = 8.6637e-01
grad_norm = 4.5926e-03
step = 16
beta = 1.6000e+01
J = 8.7438e-01
grad_norm = 4.1641e-03
step = 17
beta = 1.7000e+01
J = 8.8190e-01
grad_norm = 4.4081e-03
step = 18
beta = 1.8000e+01
J = 8.9487e-01
grad_norm = 2.5973e-03
step = 19
beta = 1.9000e+01
J = 9.0314e-01
grad_norm = 3.2183e-03
step = 20
beta = 2.0000e+01
J = 9.1222e-01
grad_norm = 2.4600e-03
[19]:
params_final = params_history[-1]
Letβs run the optimize function.
and then record the final power value (including the last iterationβs parameter updates).
Results#
First, we plot the objective function (power converted to 1st order mode) as a function of step and notice that it converges nicely!
[20]:
plt.plot(Js)
plt.xlabel("iterations")
plt.ylabel("objective function")
plt.show()
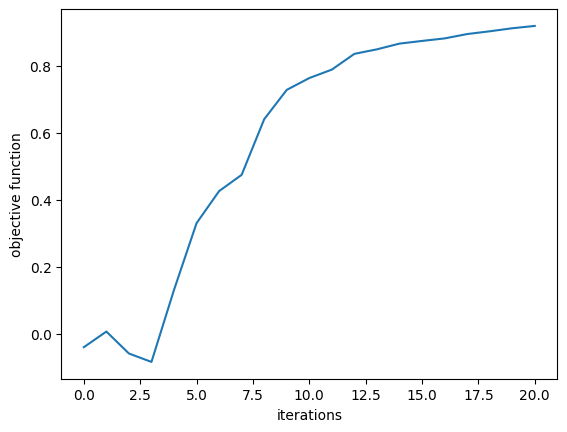
We then will visualize the final structure, so we convert it to a regular Simulation
using the final permittivity values and plot it.
[21]:
sim_final = make_sim(params_final, beta=beta)
[22]:
sim_final = sim_final.to_simulation()[0]
sim_final.plot_eps(z=0)
plt.show()
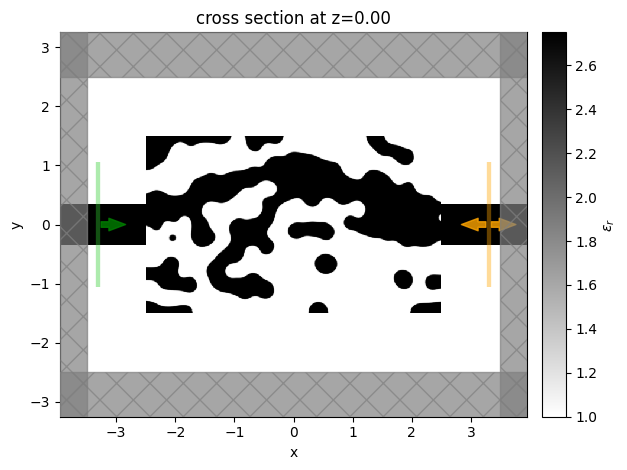
Finally, we want to inspect the fields, so we add a field monitor to the Simulation
and perform one more run to record the field values for plotting.
[23]:
field_mnt = td.FieldMonitor(
size=(td.inf, td.inf, 0),
freqs=[freq0],
name="field_mnt",
)
sim_final = sim_final.copy(update=dict(monitors=(field_mnt, measurement_monitor)))
[24]:
sim_data_final = web.run(sim_final, task_name="inv_des_final")
11:40:07 -03 Created task 'inv_des_final' with task_id 'fdve-85862bfd-ce6f-4ccb-aad9-493a31707e60' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-85862bfd-ce6 f-4ccb-aad9-493a31707e60'.
11:40:12 -03 status = queued
11:40:27 -03 status = preprocess
11:40:31 -03 Maximum FlexCredit cost: 0.025. Use 'web.real_cost(task_id)' to get the billed FlexCredit cost after a simulation run.
starting up solver
11:40:32 -03 running solver
To cancel the simulation, use 'web.abort(task_id)' or 'web.delete(task_id)' or abort/delete the task in the web UI. Terminating the Python script will not stop the job running on the cloud.
11:40:40 -03 early shutoff detected at 12%, exiting.
status = postprocess
11:40:47 -03 status = success
View simulation result at 'https://tidy3d.simulation.cloud/workbench?taskId=fdve-85862bfd-ce6 f-4ccb-aad9-493a31707e60'.
11:40:51 -03 loading simulation from simulation_data.hdf5
We notice that the behavior is as expected and the device performs exactly how we intended!
[25]:
f, (ax0, ax1, ax2) = plt.subplots(1, 3, figsize=(12, 3), tight_layout=True)
sim_final.plot_eps(z=0.01, ax=ax0)
ax1 = sim_data_final.plot_field("field_mnt", "Ez", z=0, ax=ax1)
ax2 = sim_data_final.plot_field("field_mnt", "E", "abs^2", z=0, ax=ax2)
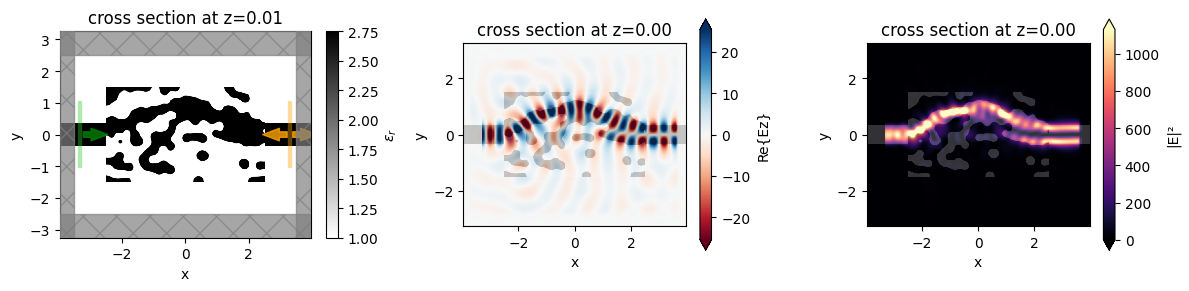
The final device converts more than 90% of the input power to the 1st mode, up from < 1% when we started.
[26]:
final_power = sim_data_final["measurement"].amps.sel(direction="+", f=freq0, mode_index=mode_index_out).abs**2
print(f"Final power conversion = {final_power*100:.2f}%")
Final power conversion = 96.06%
Export to GDS#
The Simulation
object has the .to_gds_file convenience function to export the final design to a GDS
file. In addition to a file name, it is necessary to set a cross-sectional plane (z = 0
in this case) on which to evaluate the geometry, a frequency
to evaluate the permittivity, and a permittivity_threshold
to define the shape boundaries in custom
mediums. See the GDS export notebook for a detailed example on using .to_gds_file
and other GDS related functions.
[27]:
sim_final.to_gds_file(
fname="./misc/inv_des_mode_conv.gds",
z=0,
frequency=freq0,
permittivity_threshold=2.6
)