S Parameters¶
Scattering (S) parameters describe the relationship between signals entering through the ports of a device and the signals exiting from those same ports. They are usually represented as a frequency-dependent matrix and are used to build compact models of devices in photonics, microwaves, RF, etc. In PhotonForge, the S parameters are stored as a sparse matrix in a python dictionary, as we’ll see in this guide.
Computation of the S matrix in PhotonForge is done via models, which makes it very flexible to accommodate use cases ranging from purely analytical calculations, to FDTD, or measured data. This guide shows how the S matrix computed in PhotonForge can be used for visualization and further post-processing.
Computing The S Matrix¶
We will load the Y splitter from the SiEPIC OpenEBL PDK. It includes a Tidy3D model that will leverage the power of Tidy3D to calculate the S matrix of this component using full 3D FDTD simulations.
[1]:
import numpy as np
import photonforge as pf
import siepic_forge as siepic
from matplotlib import pyplot as plt
# Set the SiEPIC technology as default in this project
pf.config.default_technology = siepic.ebeam()
[2]:
y_splitter = siepic.component("ebeam_y_1550")
y_splitter
[2]:
The actual S matrix computation requires only the specification of the simulation frequencies in the s_matrix function:
[3]:
wavelengths = np.linspace(1.5, 1.6, 11)
freqs = pf.C_0 / wavelengths
s_matrix = y_splitter.s_matrix(freqs)
Starting...
Loading cached simulation from .tidy3d/pf_cache/W34/fdtd_info-BUYNSBSWS6IRIWPZJ4GT2OCXCFTY3T7X5FC4CIN63SVJ6YUMRF6A.json.
Loading cached simulation from .tidy3d/pf_cache/W34/fdtd_info-FZCDD3BRNLGPYZRQKSVREEBCBDE77S3CJUA5DGSQPOHXN2OBUPUQ.json.
Progress: 100%
Despite the name, the returned s_matrix
object is not a matrix, but an SMatrix instance. It stores the usual S matrix elements in a dictionary and also the frequencies and ports used for the computation.
Using a dictionary instead of a multidimensional array for the elements not only alleviates memory usage in large and sparse systems, but also facilitates using port names instead of integer indices to identify the matrix elements. In this dictionary, each key is a pair of port names and mode index: (input_port_and_mode, output_port_and_mode)
. Note that this departs from the index order in electromagnetic theory, where \(S_{ij}\) represents input at \(j\)-th port and output at the
\(i\)-th port.
[4]:
s_matrix.elements.keys()
[4]:
dict_keys([('P2@0', 'P0@0'), ('P2@0', 'P1@0'), ('P0@0', 'P0@0'), ('P0@0', 'P1@0'), ('P0@0', 'P2@0'), ('P1@0', 'P0@0'), ('P2@0', 'P2@0'), ('P1@0', 'P1@0'), ('P1@0', 'P2@0')])
The port names and mode indices are joined in the s_matrix
keys with an “@” character. Because the ports used in the Y splitter are all single mode, we only have mode index 0 in our keys.
We look at the complex transmission coefficient from “P0” to “P1” for the fundamental mode: it is a Numpy array with one coefficient for each frequency/wavelength point we specified earlier.
[5]:
s_matrix[("P0@0", "P1@0")]
[5]:
array([-0.5470885 -0.4181265j , -0.31552839+0.61499621j,
0.65859861+0.21633469j, 0.12619266-0.6827587j ,
-0.69318577-0.04881665j, 0.0144139 +0.69509131j,
0.69224105-0.06279952j, -0.09594951-0.6879992j ,
-0.68494709+0.11420326j, 0.11833162+0.6840524j ,
0.68521832-0.10839435j])
We can look at the magnitude squared of those elements to get the power transmission coefficients:
[6]:
np.abs(s_matrix[("P0@0", "P1@0")]) ** 2
[6]:
array([0.47413559, 0.47777851, 0.48055282, 0.48208404, 0.48288957,
0.4833597 , 0.48314144, 0.4825492 , 0.48219491, 0.48193006,
0.48127348])
Visualization and Post-Processing¶
Post-processing and visualization is as easy as working with any Numpy arrays. For example, we can calculate the insertion loss for port P0 by subtracting the power transmission coefficients to the other ports from 1:
[7]:
trans1 = np.abs(s_matrix[("P0@0", "P1@0")]) ** 2
trans2 = np.abs(s_matrix[("P0@0", "P2@0")]) ** 2
insertion_loss = 1 - trans1 - trans2
insertion_loss
[7]:
array([0.05169103, 0.04440777, 0.03886149, 0.03580025, 0.03418974,
0.03324967, 0.03368911, 0.03487861, 0.03558866, 0.0361155 ,
0.03742654])
Plotting is just as easy too:
[8]:
_, ax = plt.subplots(1, 1)
ax.plot(wavelengths, 10 * np.log10(trans1), ".-", label="Trans. P0 → P1")
ax.plot(wavelengths, 10 * np.log10(trans2), ".-", label="Trans. P0 → P2")
ax.plot(wavelengths, 10 * np.log10(insertion_loss), ".-", label="Insertion Loss")
ax.set(xlabel="Wavelength (μm)", ylabel="Coefficient (dB)")
_ = ax.legend()
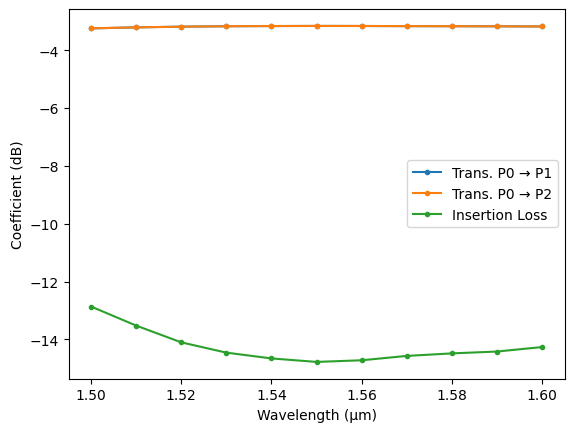
For quick visualization, PhotonForge includes the plot_s_matrix function, which can be customized to some extend to inspect the S matrix.
[9]:
_ = pf.plot_s_matrix(s_matrix)
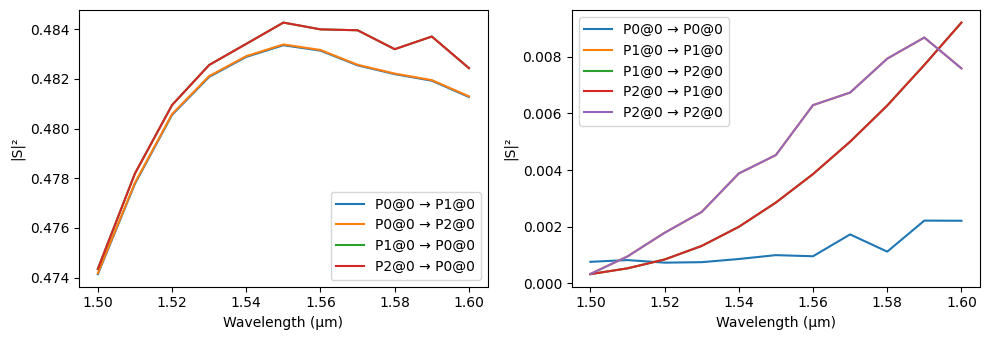
We can limit the number of input and output ports to plot, change the plot value, scale, etc.
[10]:
_ = pf.plot_s_matrix(s_matrix, x="frequency", y="phase", input_ports=["P0"], output_ports=["P1"])
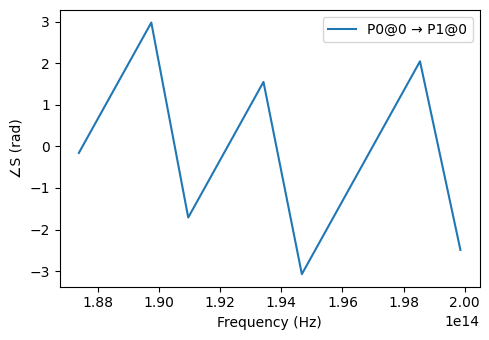