Parameter scan
Contents
Parameter scan#
Note: the cost of running the entire notebook is larger than 1 FlexUnit.
In this notebook, we will show an example of using tidy3d to evaluate device performance over a set of many design parameters.
This example will also provide a walkthrough of Tidy3D’s Job and Batch features for managing both individual simulations and sets of simulations.
For demonstration, we look at the splitting ratio of a directional coupler as we vary the coupling length between two waveguides. The sidewall of the waveguides is slanted, deviating from the vertical direction by sidewall_angle
.
[1]:
# standard python imports
import numpy as np
import matplotlib.pyplot as plt
import os
import gdstk
# tidy3D imports
import tidy3d as td
from tidy3d import web
# set tidy3d to only print error information to reduce verbosity
td.config.logging_level = "error"
[18:07:40] WARNING This version of Tidy3D was pip installed from the 'tidy3d-beta' repository on __init__.py:103 PyPI. Future releases will be uploaded to the 'tidy3d' repository. From now on, please use 'pip install tidy3d' instead.
INFO Using client version: 1.9.0rc1 __init__.py:121
Setup#
First we set up some global parameters
[2]:
# wavelength / frequency
lambda0 = 1.550 # all length scales in microns
freq0 = td.constants.C_0 / lambda0
fwidth = freq0 / 10
# Permittivity of waveguide and substrate
wg_n = 3.48
sub_n = 1.45
mat_wg = td.Medium(permittivity=wg_n**2)
mat_sub = td.Medium(permittivity=sub_n**2)
# Waveguide dimensions
# Waveguide height
wg_height = 0.22
# Waveguide width
wg_width = 0.45
# Waveguide separation in the beginning/end
wg_spacing_in = 8
# Reference plane where the cross section of the device is defined
reference_plane = "bottom"
# Angle of the sidewall deviating from the vertical ones, positive values for the base larger than the top
sidewall_angle = np.pi / 6
# Total device length along propagation direction
device_length = 100
# Length of the bend region
bend_length = 16
# space between waveguide and PML
pml_spacing = 1
# resolution control: minimum number of grid cells per wavelength in each material
grid_cells_per_wvl = 16
Define waveguide bends and coupler#
Here is where we define our directional coupler shape programmatically in terms of the geometric parameters
[3]:
def tanh_interp(max_arg):
"""Interpolator for tanh with adjustable extension"""
scale = 1 / np.tanh(max_arg)
return lambda u: 0.5 * (1 + scale * np.tanh(max_arg * (u * 2 - 1)))
def make_coupler(
length, wg_spacing_in, wg_width, wg_spacing_coup, coup_length, bend_length, npts_bend=30
):
"""Make an integrated coupler using the gdstk RobustPath object."""
# bend interpolator
interp = tanh_interp(3)
delta = wg_width + wg_spacing_coup - wg_spacing_in
offset = lambda u: wg_spacing_in + interp(u) * delta
coup = gdstk.RobustPath(
(-0.5 * length, 0),
(wg_width, wg_width),
wg_spacing_in,
simple_path=True,
layer=1,
datatype=[0, 1],
)
coup.segment((-0.5 * coup_length - bend_length, 0))
coup.segment(
(-0.5 * coup_length, 0), offset=[lambda u: -0.5 * offset(u), lambda u: 0.5 * offset(u)]
)
coup.segment((0.5 * coup_length, 0))
coup.segment(
(0.5 * coup_length + bend_length, 0),
offset=[lambda u: -0.5 * offset(1 - u), lambda u: 0.5 * offset(1 - u)],
)
coup.segment((0.5 * length, 0))
return coup
Create Simulation and Submit Job#
The following function creates a tidy3d simulation object for a set of design parameters.
Note that the simulation has not been run yet, just created.
[4]:
def make_sim(coup_length, wg_spacing_coup, domain_field=False):
"""Make a simulation with a given length of the coupling region and
distance between the waveguides in that region. If ``domain_field``
is True, a 2D in-plane field monitor will be added.
"""
# Geometry must be placed in GDS cells to import into Tidy3D
coup_cell = gdstk.Cell("Coupler")
substrate = gdstk.rectangle(
(-device_length / 2, -wg_spacing_in / 2 - 10),
(device_length / 2, wg_spacing_in / 2 + 10),
layer=0,
)
coup_cell.add(substrate)
# Add the coupler to a gdstk cell
gds_coup = make_coupler(
device_length,
wg_spacing_in,
wg_width,
wg_spacing_coup,
coup_length,
bend_length,
)
coup_cell.add(gds_coup)
# Substrate
oxide_geo, = td.PolySlab.from_gds(
gds_cell=coup_cell,
gds_layer=0,
gds_dtype=0,
slab_bounds=(-10, 0),
reference_plane=reference_plane,
axis=2,
)
oxide = td.Structure(geometry=oxide_geo, medium=mat_sub)
# Waveguides (import all datatypes if gds_dtype not specified)
coupler1_geo, coupler2_geo = td.PolySlab.from_gds(
gds_cell=coup_cell,
gds_layer=1,
slab_bounds=(0, wg_height),
sidewall_angle=sidewall_angle,
reference_plane=reference_plane,
axis=2,
)
coupler1 = td.Structure(geometry=coupler1_geo, medium=mat_wg)
coupler2 = td.Structure(geometry=coupler2_geo, medium=mat_wg)
# Simulation size along propagation direction
sim_length = 2 + 2 * bend_length + coup_length
# Spacing between waveguides and PML
sim_size = [
sim_length,
wg_spacing_in + wg_width + 2 * pml_spacing,
wg_height + 2 * pml_spacing,
]
# source
src_pos = -sim_length / 2 + 0.5
msource = td.ModeSource(
center=[src_pos, wg_spacing_in / 2, wg_height / 2],
size=[0, 3, 2],
source_time=td.GaussianPulse(freq0=freq0, fwidth=fwidth),
direction="+",
mode_spec=td.ModeSpec(),
mode_index=0,
)
mon_in = td.ModeMonitor(
center=[(src_pos + 0.5), wg_spacing_in / 2, wg_height / 2],
size=[0, 3, 2],
freqs=[freq0],
mode_spec=td.ModeSpec(),
name="in",
)
mon_ref_bot = td.ModeMonitor(
center=[(src_pos + 0.5), -wg_spacing_in / 2, wg_height / 2],
size=[0, 3, 2],
freqs=[freq0],
mode_spec=td.ModeSpec(),
name="refect_bottom",
)
mon_top = td.ModeMonitor(
center=[-(src_pos + 0.5), wg_spacing_in / 2, wg_height / 2],
size=[0, 3, 2],
freqs=[freq0],
mode_spec=td.ModeSpec(),
name="top",
)
mon_bot = td.ModeMonitor(
center=[-(src_pos + 0.5), -wg_spacing_in / 2, wg_height / 2],
size=[0, 3, 2],
freqs=[freq0],
mode_spec=td.ModeSpec(),
name="bottom",
)
monitors = [mon_in, mon_ref_bot, mon_top, mon_bot]
if domain_field == True:
domain_monitor = td.FieldMonitor(
center=[0, 0, wg_height / 2],
size=[td.inf, td.inf, 0],
freqs=[freq0],
name="field",
)
monitors.append(domain_monitor)
# initialize the simulation
sim = td.Simulation(
size=sim_size,
grid_spec=td.GridSpec.auto(min_steps_per_wvl=grid_cells_per_wvl),
structures=[oxide, coupler1, coupler2],
sources=[msource],
monitors=monitors,
run_time=20 / fwidth,
boundary_spec=td.BoundarySpec.all_sides(boundary=td.PML()),
)
return sim
Inspect Simulation#
Let’s create and inspect a single simulation to make sure it was defined correctly before doing the full scan. The sidewalls of the waveguides deviate from the vertical direction by 30 degree. We also add an in-plane field monitor to have a look at the fields evolution in this one simulation. We will not use such a monitor in the batch to avoid storing unnecesarrily large amounts of data.
[5]:
# Length of the coupling region
coup_length = 10
# Waveguide separation in the coupling region
wg_spacing_coup = 0.10
sim = make_sim(coup_length, wg_spacing_coup, domain_field=True)
[6]:
# visualize geometry
fig, (ax1, ax2) = plt.subplots(1, 2, figsize=(14, 4))
sim.plot(z=wg_height / 2 + 0.01, ax=ax1)
sim.plot(x=0.1, ax=ax2)
ax2.set_xlim([-3, 3])
[6]:
(-3.0, 3.0)
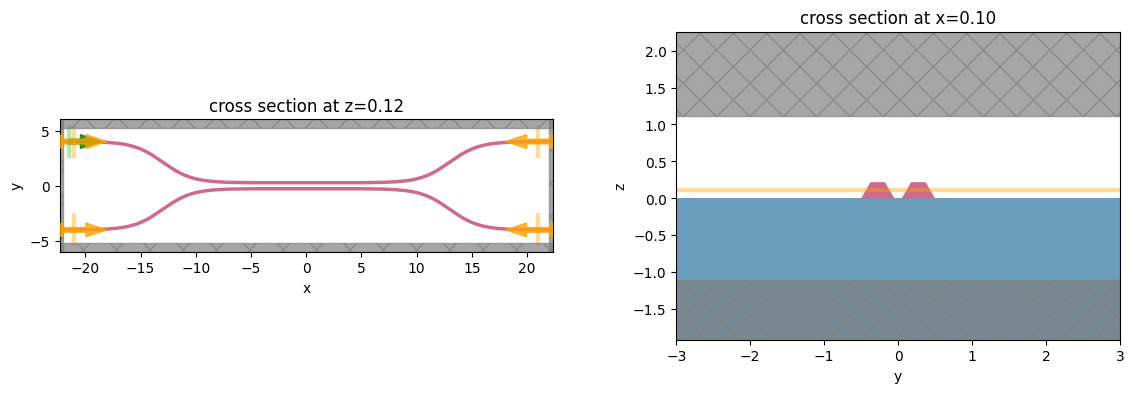
Create and Submit Job#
The Job object provides an interface for managing simulations.
job = Job(simulation)
will create a job and upload the simulation to our server to run.
Then, one may call various methods of job
to monitor progress, download results, and get information.
For more information, refer to the API reference.
[1]:
# create job, upload sim to server to begin running
job = web.Job(simulation=sim, task_name="CouplerVerify")
# download the results and load them into a simulation
sim_data = job.run(path="data/sim_data.hdf5")
---------------------------------------------------------------------------
KeyboardInterrupt Traceback (most recent call last)
Cell In[1], line 5
2 job = web.Job(simulation=sim, task_name="CouplerVerify")
4 # download the results and load them into a simulation
----> 5 sim_data = job.run(path="data/sim_data.hdf5")
File ~/Documents/Flexcompute/tidy3d-docs/tidy3d/tidy3d/web/container.py:70, in Job.run(self, path)
56 """run :class:`Job` all the way through and return data.
57
58 Parameters
(...)
66 Dictionary mapping task name to :class:`.SimulationData` for :class:`Job`.
67 """
69 self.start()
---> 70 self.monitor()
71 return self.load(path=path)
File ~/Documents/Flexcompute/tidy3d-docs/tidy3d/tidy3d/web/container.py:130, in Job.monitor(self)
122 def monitor(self) -> None:
123 """Monitor progress of running :class:`Job`.
124
125 Note
(...)
128 call :meth:`Job.load`.
129 """
--> 130 web.monitor(self.task_id)
File ~/Documents/Flexcompute/tidy3d-docs/tidy3d/tidy3d/web/webapi.py:293, in monitor(task_id)
291 new_description = f"% done (field decay = {field_decay:.2e})"
292 progress.update(pbar_pd, completed=perc_done, description=new_description)
--> 293 time.sleep(1.0)
294 if perc_done is not None and perc_done < 100:
295 log.info("early shutoff detected, exiting.")
KeyboardInterrupt:
Postprocessing#
The following function takes a completed simulation (with data loaded into it) and computes the quantities of interest.
For this case, we measure both the total transmission in the right ports and also the ratio of power between the top and bottom ports.
[8]:
def measure_transmission(sim_data):
"""Constructs a "row" of the scattering matrix when sourced from top left port"""
input_amp = sim_data["in"].amps.sel(direction="+")
amps = np.zeros(4, dtype=complex)
directions = ("-", "-", "+", "+")
for i, (monitor, direction) in enumerate(
zip(sim_data.simulation.monitors[:4], directions)
):
amp = sim_data[monitor.name].amps.sel(direction=direction)
amp_normalized = amp / input_amp
amps[i] = np.squeeze(amp_normalized.values)
return amps
[9]:
# monitor and test out the measure_transmission function the results of the single run
amps_arms = measure_transmission(sim_data)
print("mode amplitudes in each port:\n")
for amp, monitor in zip(amps_arms, sim_data.simulation.monitors[:-1]):
print(f'\tmonitor = "{monitor.name}"')
print(f"\tamplitude^2 = {abs(amp)**2:.2f}")
print(f"\tphase = {(np.angle(amp)):.2f} (rad)\n")
mode amplitudes in each port:
monitor = "in"
amplitude^2 = 0.00
phase = -0.18 (rad)
monitor = "refect_bottom"
amplitude^2 = 0.00
phase = -2.06 (rad)
monitor = "top"
amplitude^2 = 0.81
phase = 2.06 (rad)
monitor = "bottom"
amplitude^2 = 0.17
phase = -2.65 (rad)
[10]:
fig, ax = plt.subplots(1, 1, figsize=(16, 3))
# sim_data['field'].Ey.real.interp(z=0).plot()
sim_data.plot_field("field", "Ey", z=wg_height / 2, freq=freq0, ax=ax)
[10]:
<AxesSubplot: title={'center': 'cross section at z=0.11'}, xlabel='x', ylabel='y'>
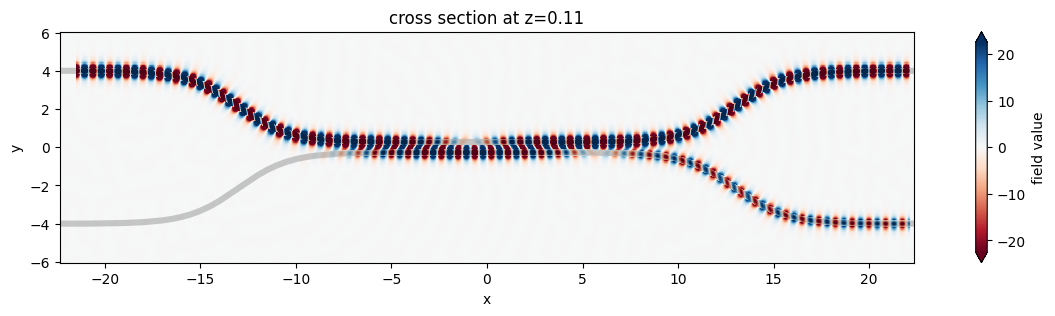
1D Parameter Scan#
Now we will scan through the coupling length parameter to see the effect on splitting ratio.
To do this, we will create a list of simulations corresponding to each parameter combination.
We will use this list to create a Batch object, which has similar functionality to Job but allows one to manage a set of jobs.
First, we create arrays to store the input and output values.
[11]:
# create variables to store parameters, simulation information, results
Nl = 11
ls = np.linspace(5, 12, Nl)
split_ratios = np.zeros(Nl)
efficiencies = np.zeros(Nl)
Create Batch#
We now create our list of simulations and use them to initialize a Batch.
For more information, refer to the API reference.
[12]:
# submit all jobs
sims = {f"l={l:.2f}": make_sim(l, wg_spacing_coup) for l in ls}
batch = web.Batch(simulations=sims)
Monitor Batch#
Here we can perform real-time monitoring of how many of the jobs in the batch have completed.
[13]:
batch_results = batch.run(path_dir="data")
[19:47:20] Started working on Batch. container.py:361
[19:51:47] Batch complete. container.py:382
Load and visualize Results#
Finally, we can compute the output quantities and load them into the arrays we created initally.
Then we may plot the results.
[14]:
amps_batch = []
for task_name, sim_data in batch_results.items():
amps_arms_i = np.array(measure_transmission(sim_data))
amps_batch.append(amps_arms_i)
amps_batch = np.stack(amps_batch, axis=1)
print(amps_batch.shape) # (4, Nl)
print(amps_batch)
(4, 11)
[[ 4.61903757e-04-3.02491013e-03j -1.76492290e-03-1.93131155e-03j
1.51192371e-03-8.42259248e-04j 6.24655075e-04-3.53510876e-03j
-1.30511215e-03-5.56092026e-04j -1.97910271e-07-1.58548088e-03j
-6.10997873e-04-1.21667803e-03j 2.51348630e-03-2.68261448e-03j
-1.74108484e-03-9.25419904e-04j 2.70415809e-03-2.75713135e-03j
-1.45349968e-03-2.00091314e-03j]
[-1.32584887e-03-5.84756253e-04j -1.67244170e-03+9.38134945e-04j
-1.22111001e-03+1.63926002e-03j -1.73482547e-03+1.15987476e-03j
-1.14609934e-03-1.16893477e-04j -2.85371434e-03+2.19864195e-03j
6.33296659e-04+7.68090523e-04j -4.54542595e-03+1.11685248e-03j
1.44825955e-03+1.89316216e-03j -3.06304615e-03+8.07302639e-04j
-1.06741732e-03+8.34135854e-04j]
[-6.49056719e-01-3.22110390e-01j -5.82842879e-01+5.85609355e-01j
3.74286731e-01+8.24815394e-01j 9.58552261e-01-6.42287299e-02j
2.82465450e-01-9.47960619e-01j -7.95310568e-01-5.90397933e-01j
-8.06808581e-01+5.28963845e-01j 2.32159738e-01+8.82190740e-01j
8.30079552e-01+8.37257217e-02j 3.18502709e-01-6.60627874e-01j
-4.18337818e-01-4.47732829e-01j]
[-3.01396004e-01+6.07863020e-01j 3.88921754e-01+3.88173917e-01j
3.69797256e-01-1.67179244e-01j -1.65351864e-02-2.50688913e-01j
-8.58314801e-02-2.54214021e-02j 4.51525150e-02-5.99073311e-02j
-1.29646668e-01-1.99187133e-01j -3.81229943e-01+9.96459514e-02j
-5.47072505e-02+5.36804310e-01j 6.03151006e-01+2.91214000e-01j
5.70751230e-01-5.33449609e-01j]]
[15]:
powers = abs(amps_batch) ** 2
power_top = powers[2]
power_bot = powers[3]
power_out = power_top + power_bot
[16]:
plt.plot(ls, 100 * power_top, label="Top")
plt.plot(ls, 100 * power_bot, label="Bottom")
plt.plot(ls, 100 * power_out, label="Top + Bottom")
plt.xlabel("Coupling length (µm)")
plt.ylabel("Power ratio (%)")
plt.ylim(0, 100)
plt.legend()
[16]:
<matplotlib.legend.Legend at 0x12de69210>
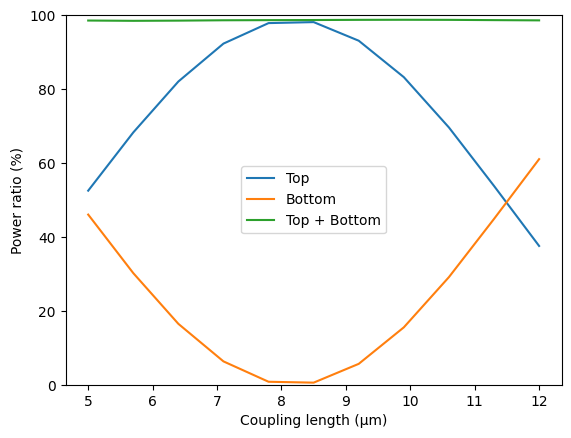
Final Remarks#
Batches provide some other convenient functionality for managing large numbers of jobs.
For example, one can save the batch information to file and load the batch at a later time, if needing to disconnect from the service while the jobs are running.
[17]:
# save batch metadata
batch.to_file("data/batch_data.json")
# load batch metadata into a new batch
loaded_batch = web.Batch.from_file("data/batch_data.json")
For more reference, refer to our documentation.