3.2. Automated Meshing
3.2.1. Overview
Flow360 offers automated meshing, from CAD geometry to a surface mesh and finally to a volume mesh. The supported CAD format is *.csm file which is Engineering Sketch Pad (EPS) format. The generated volume format is CGNS.
3.2.2. Geometry
The Engineering Sketch Pad is a solid-modeling, feature-based, web-enabled system for building parametric geometry. It can be downloaded from ESP’s website. Please check out pre-built ESP, you don’t need to compile software from source. The geometry in ESP is described in a text *.csm file containing all CAD instructions. See csm example:
# Branches:
sphere 0 0 0 1
attribute groupName $mysphere
end
which will create a sphere of radius = 1 at (0, 0, 0). The face of the sphere will be labelled as mysphere.
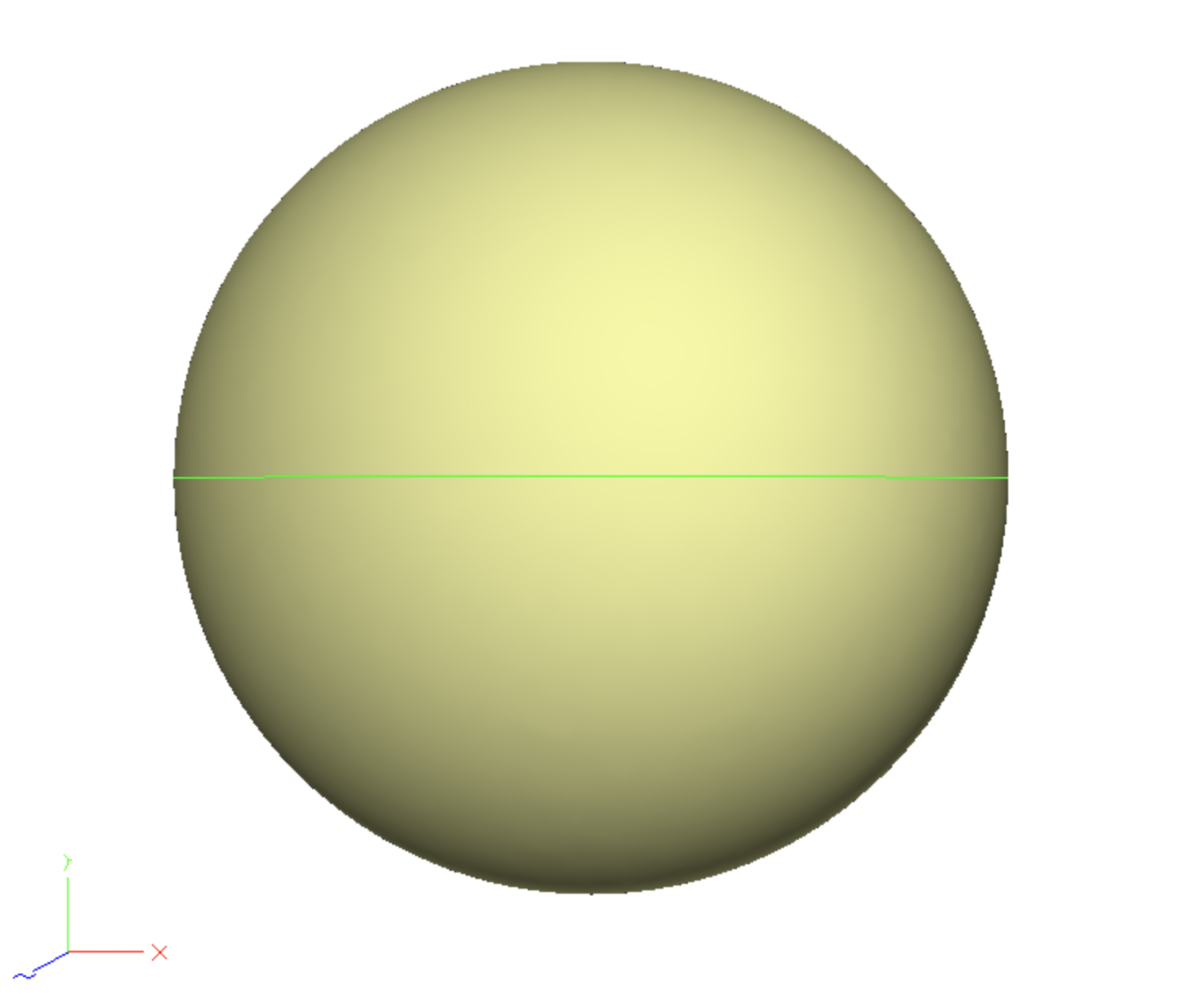
Fig. 3.2.1 Engineering Sketch Pad view of the geometry.
3.2.3. Surface Meshing
The surface mesher takes the geometry file and configuration JSON file as input parameters and generates a surface mesh. The meshing configuration file (or dict in python), also called surfaceMesh.json, contains information such as maximum element edge length, curvature resolution angle or growth rate of 2D layers. See JSON example:
{
"maxEdgeLength": 0.1,
"curvatureResolutionAngle": 15,
"growthRate": 1.2,
"faces": {
"mysphere": {
"maxEdgeLength": 0.05,
"adapt": false
}
}
}
The surface mesh is created by submitting a geometry file and JSON file using NewSurfaceMeshFromGeometry()
function. See the example below:
import flow360client
surfaceMeshId = flow360client.NewSurfaceMeshFromGeometry("path/to/geometry.csm", "surfaceMesh.json", surfaceMeshName="my_surface_mesh", solverVersion='release-21.4.1.0')
The above code will create a surface mesh of geometry using "maxEdgeLength": 0.05
on a surface labelled as mysphere, see line 4 of the *.csm file.
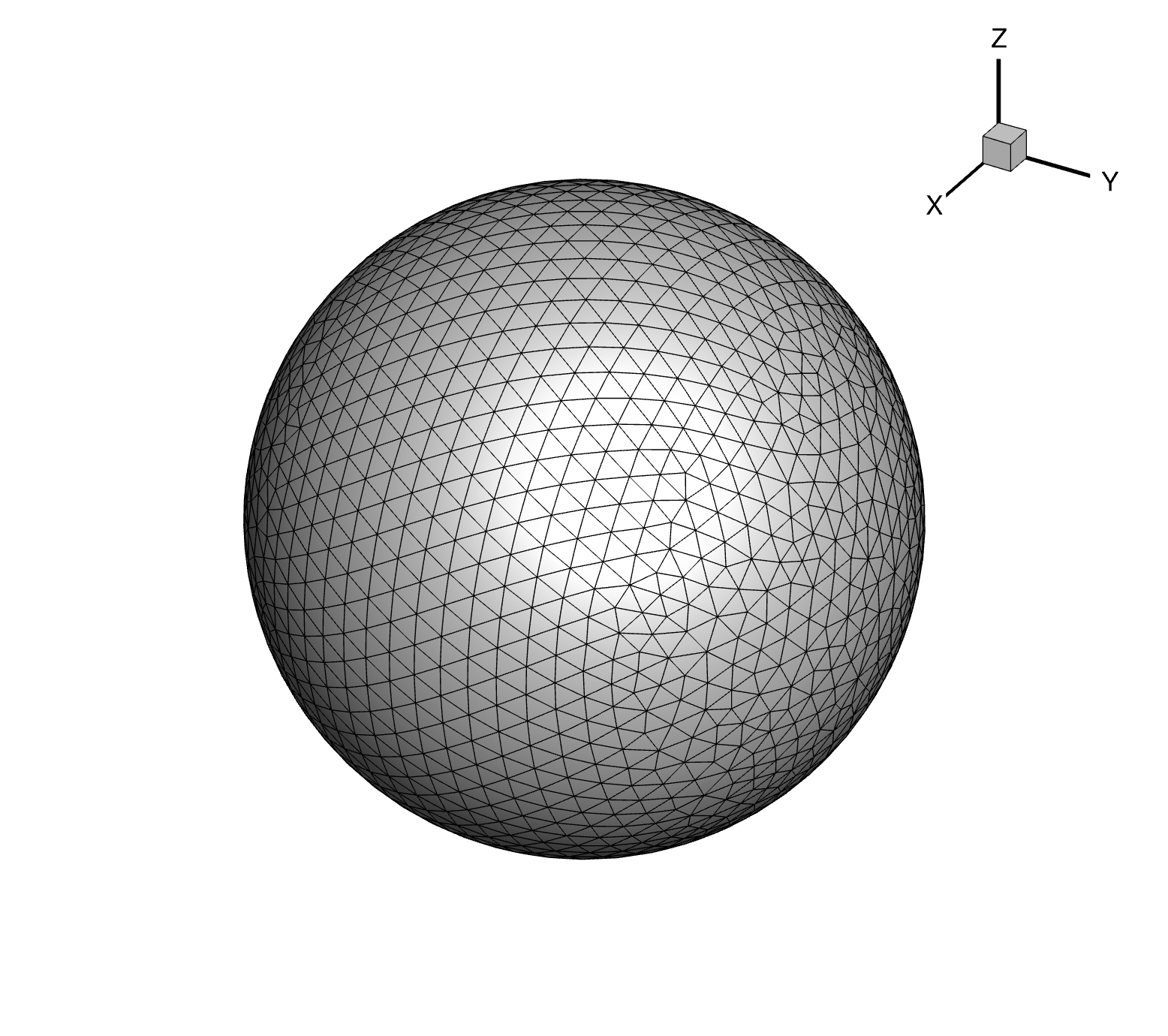
Fig. 3.2.2 Auto-generated surface mesh.
Inputs:
geometry.csm file
surface mesh config.json or python dict
Outputs:
surface mesh
surfaceMeshId
For a full description of config.json for surface mesher see here.
Note
When using web interface make sure to select version during New Surface Mesh upload
3.2.4. Volume Meshing
The volume mesher takes a surfaceMeshId
and config JSON as arguments and generates a CGNS mesh suitable for the Flow360 solver. The JSON configuration file (or dict in python) specifies the first layer thickness, growth rate, sizes and location of refinement zones (sources) and actuator disks. All geometry from ESP is treated as a no-slip wall therefore prism layers will be grown off geometry surfaces.
The farfield will be created automatically:
semi-spherical if the geometry is bounded by
y=0
plane. They=0
plane will be set as a symmetry plane boundary condition.spherical otherwise.
Below is an example of a JSON configuration file:
{
"sources": [
{
"size": [4, 3, 2],
"axisOfRotation": [ 0, 0, 1 ],
"angleOfRotation": 45,
"center": [2, 0, 0],
"spacing": 0.05
}
],
"volume": {
"firstLayerThickness": 1e-3,
"TRexGrowthRate": 1.2
}
}
The volume mesh is created by NewMeshFromSurface()
function using surfaceMeshId
and config.json. See the example below:
volumeMeshId = flow360client.NewMeshFromSurface(surfaceMeshId, "volumeMesh.json", meshName="my_volume_mesh")
The above code will create a volume mesh out of the surface mesh. The refinement zone (source) of size=4x3x2 will be placed with its centre at (2,0,0). Additionally, it will be rotated by 45 degrees around the [0,0,1] axis. Spacing of 0.05 will be applied in this zone.
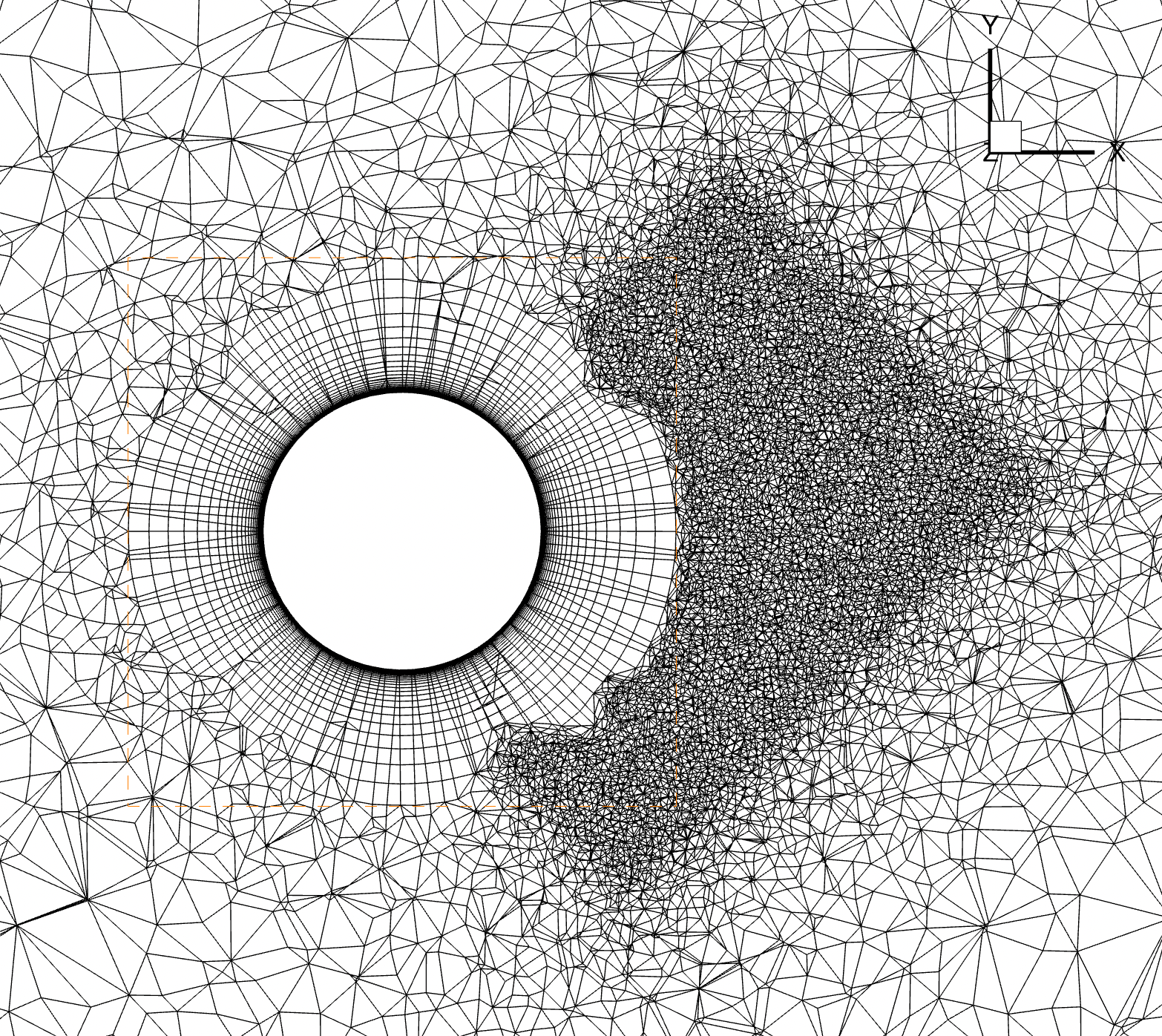
Fig. 3.2.3 Auto-generated volume mesh.
Inputs:
surfaceMeshId
volume mesh config.json or python dict
Outputs:
volume CGNS mesh
Flow360Mesh.json
volumeMeshId
For a full description of config.json for volume mesher see here.
3.2.5. JSON surface mesher
Option |
Type |
Default |
Example |
Description |
---|---|---|---|---|
maxEdgeLength |
float |
REQUIRED |
0.1 |
Maximum length of element edge. |
curvatureResolutionAngle |
float |
REQUIRED |
15 |
Maximum angular deviation in degrees. If the angle between the normal line segment and the normal to the underlying geometry curve at a grid point is larger than the specified angle, a new grid point will be added in the middle of the segment. |
growthRate |
float |
REQUIRED |
1.2 |
Growth rate of layers being grown from edges |
edges |
dict |
{} |
<edgeName>: {…} sets parameters for the edge named <edgeName>. The edge must be labelled in ESP (*.csm file). For details see here. |
|
faces |
dict |
{} |
<faceName>: {…} sets parameters for the face named <faceName>. The face must be labelled in ESP (*.csm file). For details see here. |
3.2.5.1. edges
Option |
Type |
Default |
Example |
Description |
---|---|---|---|---|
<edgeName>-> firstLayerThickness |
float |
REQUIRED |
1e-3 |
First layer thickness of the anisotropic surface cells growing along the orthogonal direction to the edge named <edgeName>. See Fig. 3.2.4. |
Example:
"edges": {
"leadingEdge": {
"firstLayerThickness": 1e-3
},
"trailingEdge": {
"firstLayerThickness": 1e-3
}
}
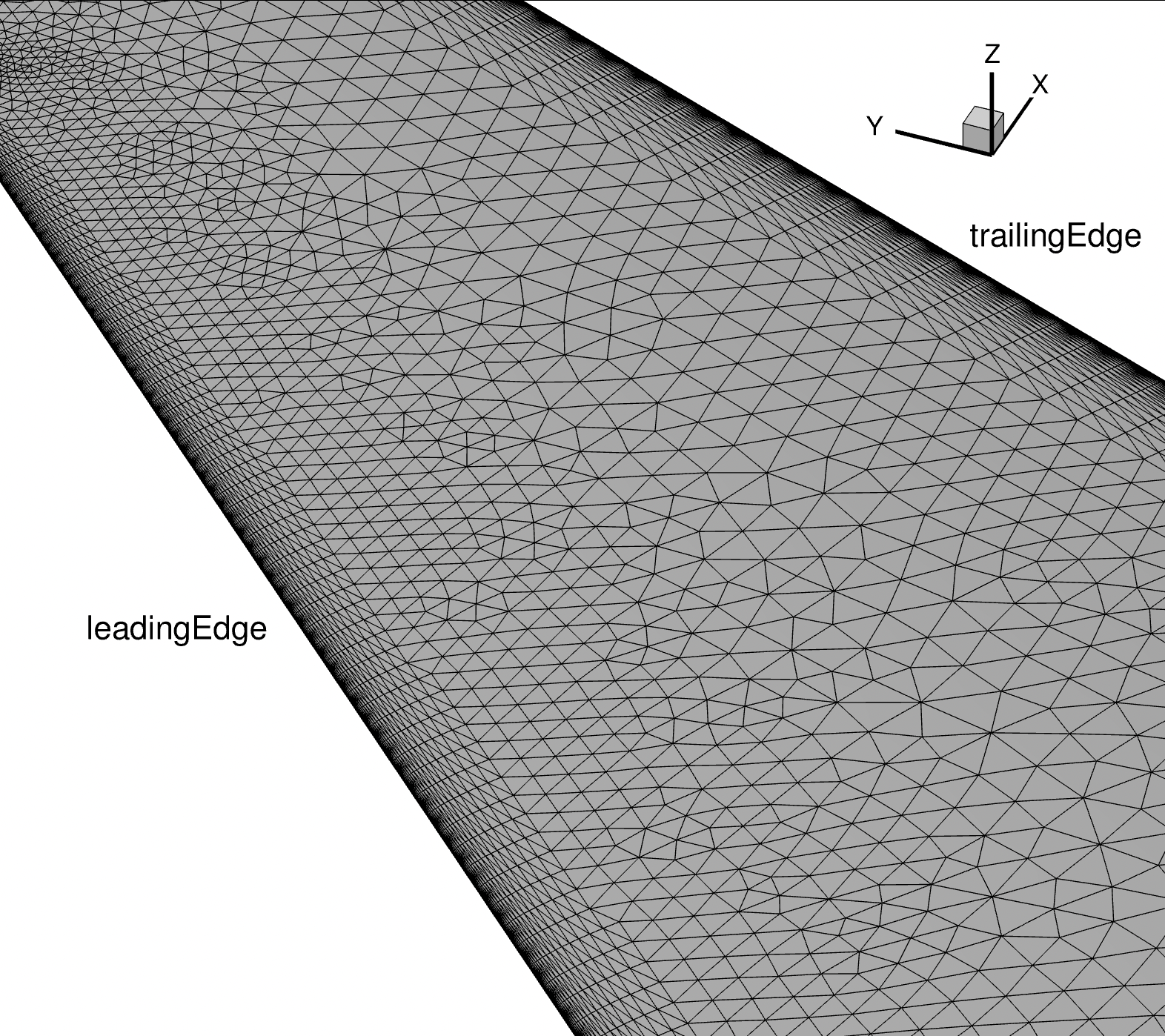
Fig. 3.2.4 Surface mesh of a wing. Leading edge and trailing edge are labelled and first layer thickness is applied to the layers grown from the edges.
3.2.5.2. faces
Option |
Type |
Default |
Example |
Description |
---|---|---|---|---|
<faceName>-> maxEdgeLength |
float |
REQUIRED |
0.05 |
Maximum length of element edge for the face named <faceName>. |
<faceName>-> adapt |
boolean |
REQUIRED |
FALSE |
Turn on/off the extra refinement based on surface proximity. [This argument will be removed in the next release and the proximity refinement will be automatically decided.] |
Example:
"faces": {
"rightWing": {
"maxEdgeLength": 0.05,
"adapt": false
},
"fuselage": {
"maxEdgeLength": 0.05,
"adapt": false
}
}
3.2.6. JSON volume mesher
Option |
Type |
Default |
Example |
Description |
---|---|---|---|---|
refinementFactor |
float |
1 |
2 |
If refinementFactor=r is provided all spacings will be adjusted to generate r-times finer mesh everywhere. For example, if refinementFactor=2, all spacings will be divided by \(2^{1/3}\approx 1.26\) , so the resulting mesh will have approximately 2 times more nodes. |
volume |
dict |
REQUIRED |
First layer thickness and growth rate for prism layers being grown out of the surface. It is applied to all surface mesh faces. |
|
volume-> firstLayerThickness |
float |
REQUIRED |
1e-5 |
First layer thickness for volume prism layers. |
volume-> TRexGrowthRate |
float |
REQUIRED |
1.2 |
Growth rate for volume prism layers. |
sources |
list(dict) |
[] |
Description of refinement zones. For details see here. |
|
actuatorDisks |
list(dict) |
[] |
Description of actuator disks. This generates a cylindrical shape (hollowed or not) with structured mesh in it. Used for actuator disks. For details see here. |
3.2.6.1. sources (list)
Option |
Type |
Default |
Example |
Description |
---|---|---|---|---|
type |
[“box”, “cylinder”] |
“box” |
“box” |
Type of source. Supported source shapes: box and cylinder. Cylinder’s axis is along the z-direction. |
size |
3-array |
REQUIRED |
[4, 3, 2] |
For type: box. Refinement zone size in x, y and z directions. |
radius |
float |
REQUIRED |
4 |
For type: cylinder. Radius of the cylinder. |
lengthZ |
float |
REQUIRED |
5 |
For type: cylinder. Length of the cylinder. Cylinder’s axis is along the z-direction. |
center |
3-array |
REQUIRED |
[2, 0, 0] |
Position of geometrical center of the primitive, i.e., box or cylinder. |
spacing |
float |
REQUIRED |
0.05 |
Cell spacing applied in the refinement zone. |
axisOfRotation |
3-array |
[0, 0, 1] |
Axis of rotation for rotation transform of the primitive (box or cylinder). Requires angleOfRotation. |
|
angleOfRotation |
float |
45 |
The angle of rotation (in degrees) for rotation transform of the primitive (box or cylinder). Requires axisOfRotation. |
Example:
"sources": [
{
"size": [4, 3, 2],
"center": [2, 0, 0],
"spacing": 0.05,
"axisOfRotation": [ 0, 0, 1 ],
"angleOfRotation": 45
},
{
"type": "cylinder",
"radius": 4,
"lengthZ": 5,
"center": [5, 0, 0],
"spacing": 0.05,
"axisOfRotation": [0, 1, 0],
"angleOfRotation": 90
}]
3.2.6.2. actuatorDisks (list)
Option |
Type |
Default |
Example |
Description |
---|---|---|---|---|
innerRadius |
float |
REQUIRED |
0 |
Inner radius of actuator disk, if greater than 0 then “donut” shape is created. |
outerRadius |
float |
REQUIRED |
10 |
Outer radius of actuator disk. |
thickness |
float |
REQUIRED |
0.5 |
Thickness of actuator disk. |
axisThrust |
3-array |
REQUIRED |
[1, 0, 0] |
Axis of thrust of actuator disk. The cylindrical shape is rotated such its axis is aligned with axisThrust. |
center |
3-array |
REQUIRED |
[0, 0, 0] |
Position of center of the actuator disk. |
spacingAxial |
float |
REQUIRED |
0.01 |
Spacing along the axial direction. |
spacingRadial |
float |
REQUIRED |
0.03 |
Spacing along the radial direction. |
spacingCircumferential |
float |
REQUIRED |
0.03 |
Spacing along the circumferential direction. |
Example:
"actuatorDisks": [
{
"innerRadius": 0,
"outerRadius": 10,
"thickness": 0.5,
"axisThrust": [1, 0, 0],
"center": [0, 0, 0],
"spacingAxial": 0.01,
"spacingRadial": 0.03,
"spacingCircumferential": 0.03
}]